top of page
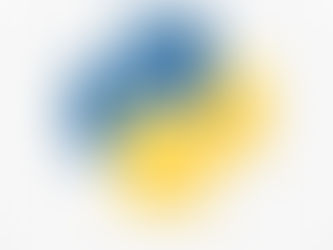
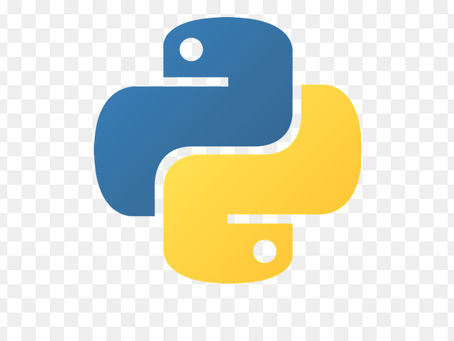
Set in Python
The set data type is supported in Python. There are only distinct elements in a set. a = {"new", "old", 1, 2} print(a) print(len(a)) The...
Apr 9, 20231 min read
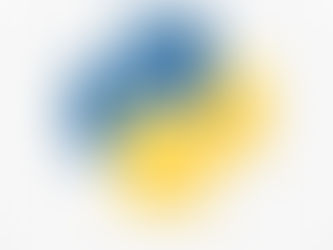
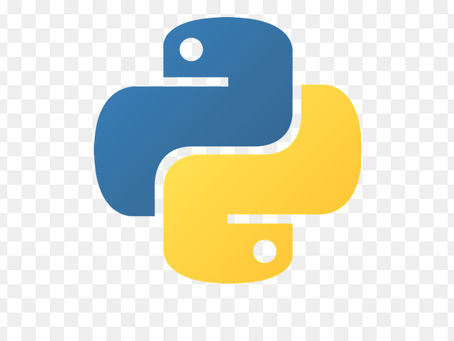
Print method in Python
The print() method is used to print the values in a console. a = 200 print(a) b = 300 print(a,b) We can also use arithmetic operators to...
Apr 9, 20231 min read
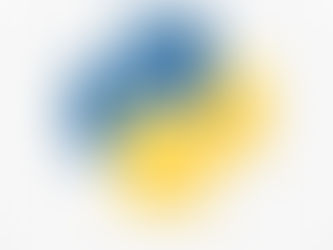
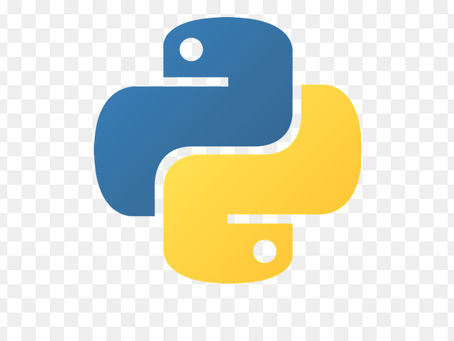
Assigning multiple variables at once in Python
We can assign values to multiple variables by passing comma separated values as shown below: a, b, c = 10, 20, "Hello World!" print(a)...
Apr 9, 20231 min read
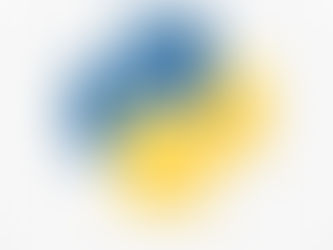
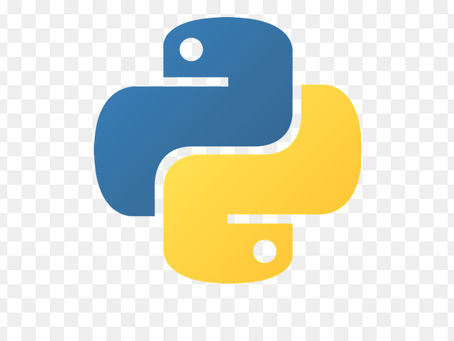
Loops in Python
There are two types of loops namely for and while. a = [1,2,3,4] for x in a: print(x) i = 0 while i < len(a): i = i + 1 if i == 2:...
Apr 9, 20231 min read
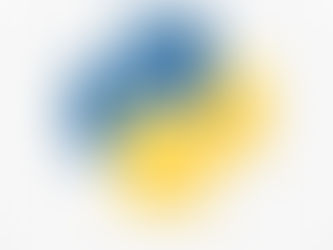
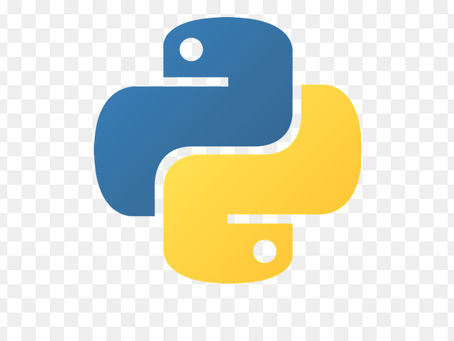
Lists in Python
Lists are like arrays. A list can have elements of any data type. a = [10,20,30,40,50, "ABC", True, 2.09, 3j] print(a) The len() is used...
Apr 9, 20232 min read
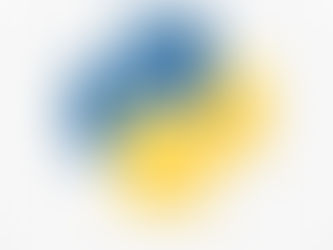
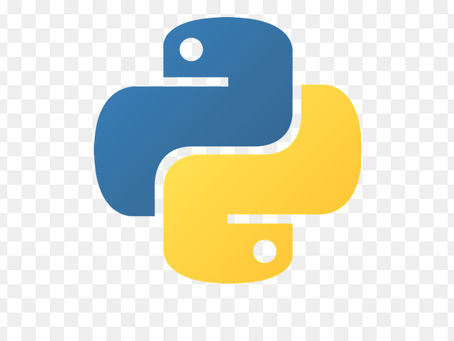
Lambda Functions in Python
Lambda function is nothing but a small anonymous function having only one expression. We can pass any number of arguments to a lambda...
Apr 9, 20231 min read
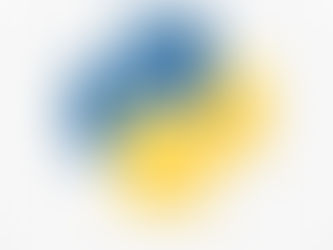
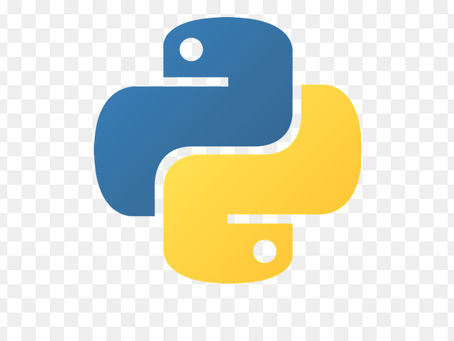
Inheritance in Python
Python supports inheritance. The parent class properties and methods are inherited by the child class. class Teacher: def __init__(self,...
Apr 9, 20231 min read
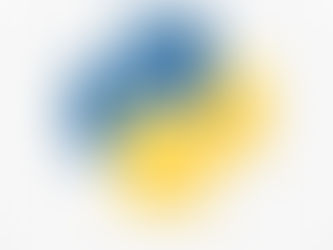
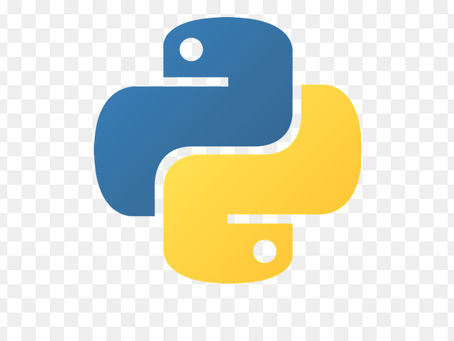
If-Else Statement in Python
If statement is used to check whether a condition is either true or not. If a condition is false, then the elif statement is used to...
Apr 9, 20231 min read
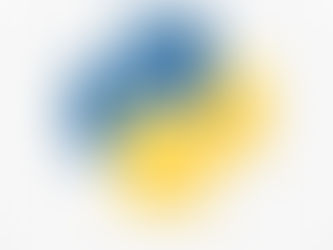
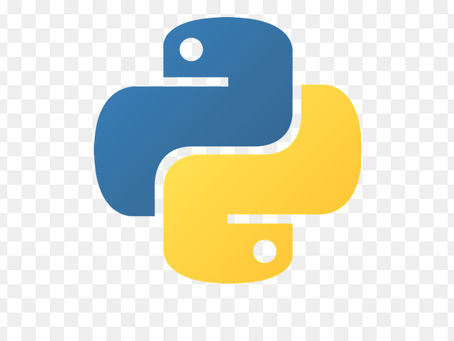
Global Variables in Python
The global variables can be used throughout the program. a = 100 def fun1(): print('Number is:', a) fun1() If the global variable is...
Apr 9, 20231 min read
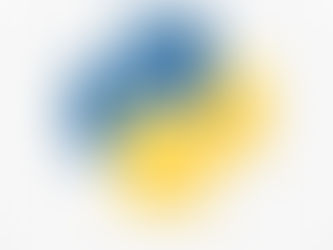
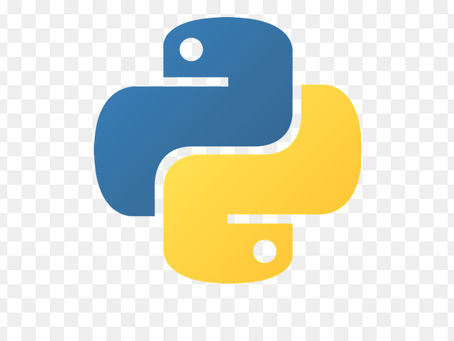
Functions in Python
Functions are small units of code that carry out specific functionalities as defined by the user. The syntax is def followed by the...
Apr 9, 20231 min read
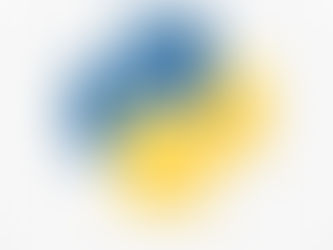
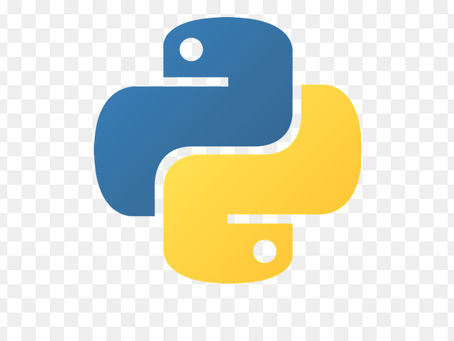
File Operations in Python
File operations in Python are simple as seen in the following example. The open() method is used to open a file. There are several...
Apr 9, 20231 min read
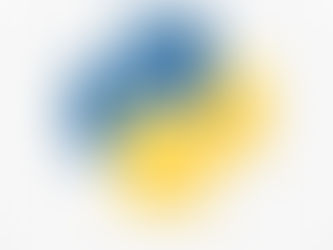
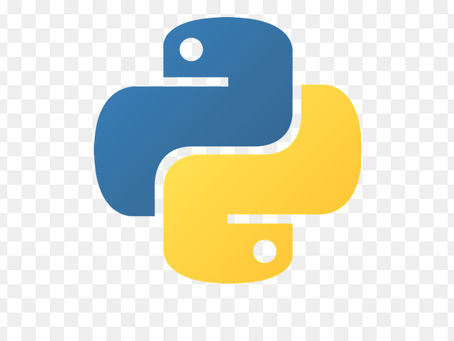
Dictionaries in Python
Python supports dictionaries. They are key-value pairs. Keys are unique. Values can be any data type. a = { "cycle": "ABC", "year": 1999 }...
Apr 9, 20231 min read
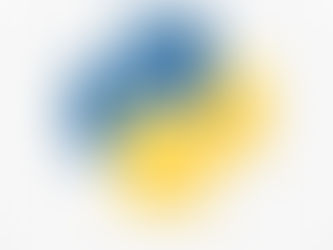
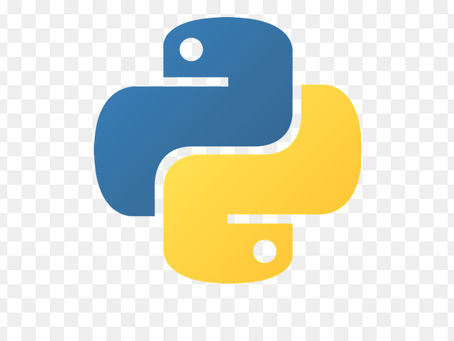
Python Datatypes
Python supports several data types. They are string, int, float, list, tuple, range, dict, set, frozenset, bool, bytes, bytearray,...
Apr 8, 20231 min read
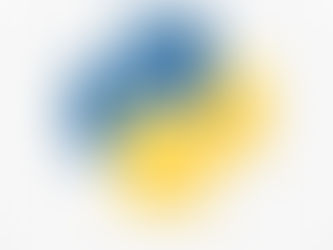
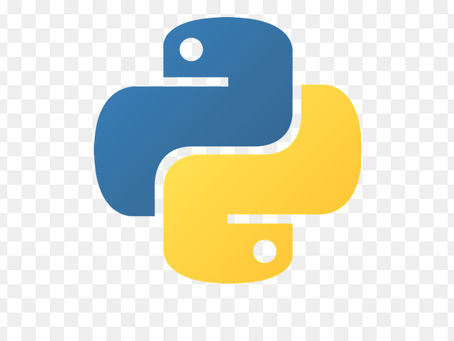
String concatenation in Python
Strings in Python can be concatenated or joined in a simple way. We can use the + sign to concat two strings. x = "Metric" y = "Coder" ...
Apr 8, 20231 min read
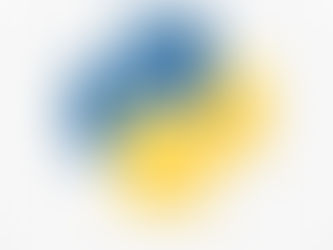
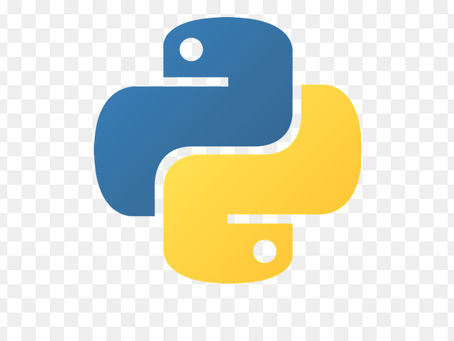
Classes in Python
Everything in Python is just like an object. A class comprises methods and attributes or properties. In the following example, we have...
Apr 8, 20231 min read
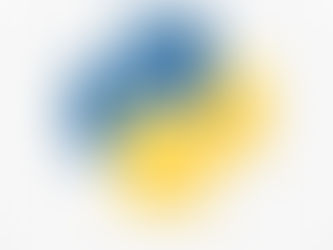
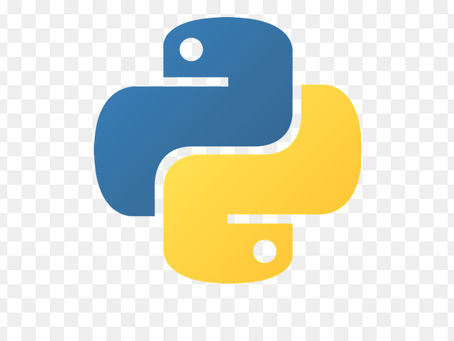
Python Comments
A comment is a non executable line of code. There are two types of comments in Python. They are single line comments and multi-line...
Apr 8, 20231 min read
bottom of page