How to Build an AI Agent Using a Large Language Model (LLM)
- Metric Coders
- Jan 20
- 3 min read
Updated: Jan 25
Artificial intelligence (AI) has taken the tech world by storm, and one of its most exciting advancements is the rise of large language models (LLMs). Models like OpenAI’s GPT have revolutionized how we think about language processing, enabling applications in customer support, content creation, automation, and more. But how do you go from a powerful LLM to a fully functional AI agent? Let’s break it down.
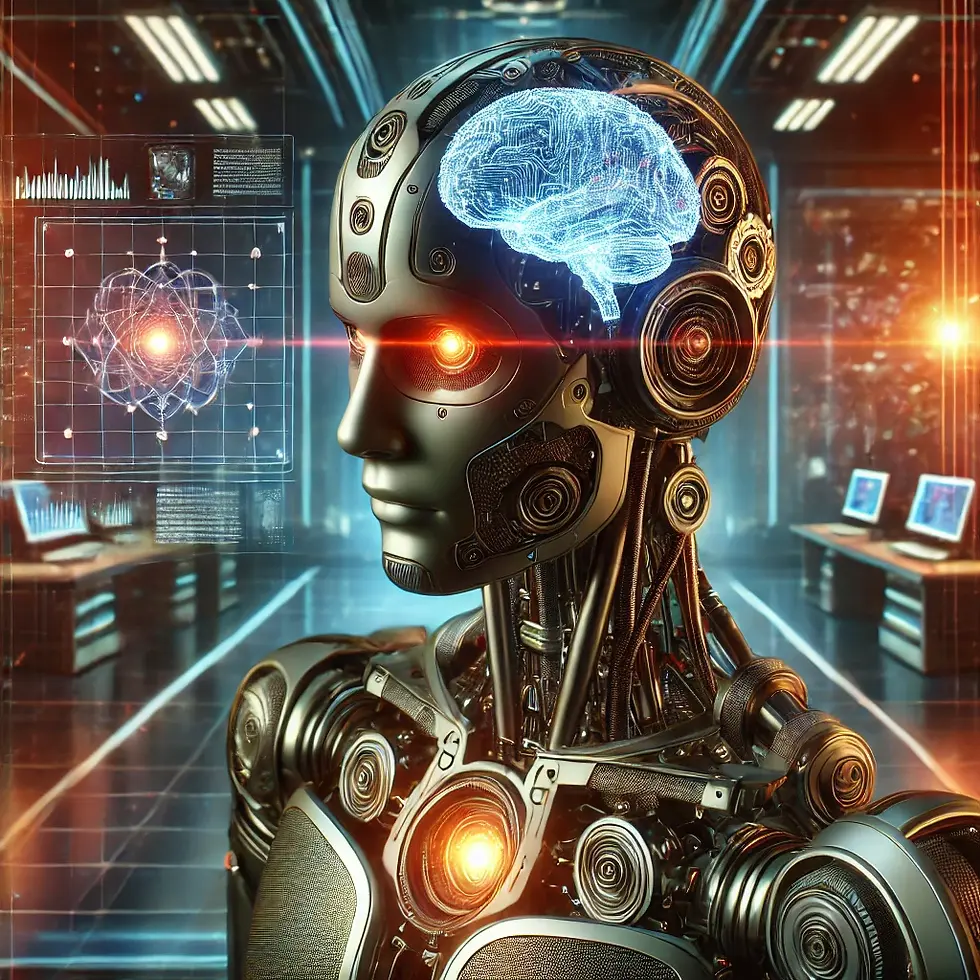
What is an AI Agent?
An AI agent is a system that can perceive its environment, process inputs, and perform actions autonomously to achieve a specific goal. When powered by an LLM, it becomes capable of understanding and generating human-like text, making it incredibly versatile and interactive.
Some examples of AI agents include:
Chatbots for customer support.
Virtual assistants for scheduling or task automation.
Tools for summarizing large datasets or documents.
Content creators that draft articles, scripts, or reports.
Steps to Build an AI Agent with an LLM
1. Define the Agent’s Purpose
Before diving into code, ask yourself: what do you want your agent to do? Define its core functionality, audience, and scope. For instance, will it answer FAQs, automate data analysis, or act as a creative writing assistant?
2. Choose the Right LLM
Several LLMs are available, each with unique capabilities. Some popular options include:
OpenAI’s GPT-4: General-purpose, powerful, and developer-friendly.
Anthropic’s Claude: Focused on safety and interpretability.
Google’s PaLM: Optimized for multilingual and multi-modal tasks.
Hugging Face Models: Open-source options for custom fine-tuning.
Select a model based on your requirements and budget. For sensitive or highly specific tasks, consider fine-tuning an open-source model.
3. Set Up the Development Environment
You’ll need a programming environment to interact with the LLM. Popular languages like Python offer robust libraries and APIs for working with LLMs.
Basic setup:
Install Python.
Use tools like Jupyter Notebook or Visual Studio Code for coding.
Install necessary libraries, such as OpenAI’s SDK (openai), Hugging Face Transformers, or LangChain.
4. Integrate the LLM
Connect to the LLM’s API to start sending and receiving text-based prompts. Here’s a basic example with OpenAI:
import openai
# Set up your API key
openai.api_key = "your_api_key_here"
# Send a prompt
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "user", "content": "Hello, what can you do?"}]
)
# Print the response
print(response['choices'][0]['message']['content'])
5. Implement Agent Logic
Your AI agent needs logic to interact with users or other systems. Use frameworks like LangChain or custom scripts to:
Process user input.
Handle specific tasks like database queries or API calls.
Maintain context during conversations.
For instance, an agent answering questions about products might:
Parse user queries.
Retrieve relevant data from a database.
Generate responses using the LLM.
6. Add Memory and Context
For an AI agent to feel intelligent, it should maintain context during interactions. You can achieve this by storing conversation history and feeding it back to the LLM in subsequent prompts. Here’s an example:
conversation = []
# Add initial user message
conversation.append({"role": "user", "content": "Tell me about AI agents."})
# Send the conversation history to the LLM
response = openai.ChatCompletion.create(
model="gpt-4",
messages=conversation
)
# Add the LLM's response to the conversation history
conversation.append({"role": "assistant", "content": response['choices'][0]['message']['content']})
print(conversation)
7. Deploy Your AI Agent
Once your agent is functional, it’s time to deploy it. Common deployment options include:
Web apps: Use frameworks like Flask or Django to build a user interface.
Messaging platforms: Integrate with platforms like Slack, WhatsApp, or Discord.
Custom APIs: Build a backend API that others can connect to.
Cloud platforms like AWS, Azure, and Google Cloud can help scale your deployment to handle more users.
8. Test and Iterate
AI agents require rigorous testing to ensure they perform reliably. Monitor their behavior, collect user feedback, and fine-tune responses to improve the experience.
Best Practices for Building AI Agents
Focus on User Experience: Ensure your agent is easy to interact with and provides helpful responses.
Handle Edge Cases: Plan for scenarios where the agent might not know the answer or misunderstand inputs.
Maintain Ethical Standards: Avoid generating harmful or biased content.
Optimize Costs: Minimize API usage by batching requests or fine-tuning smaller models.
Conclusion
Building an AI agent using a large language model can be both rewarding and transformative. With the right tools and a clear purpose, you can create agents that enhance productivity, delight users, and unlock new possibilities for automation. Start small, iterate often, and watch your AI agent come to life!